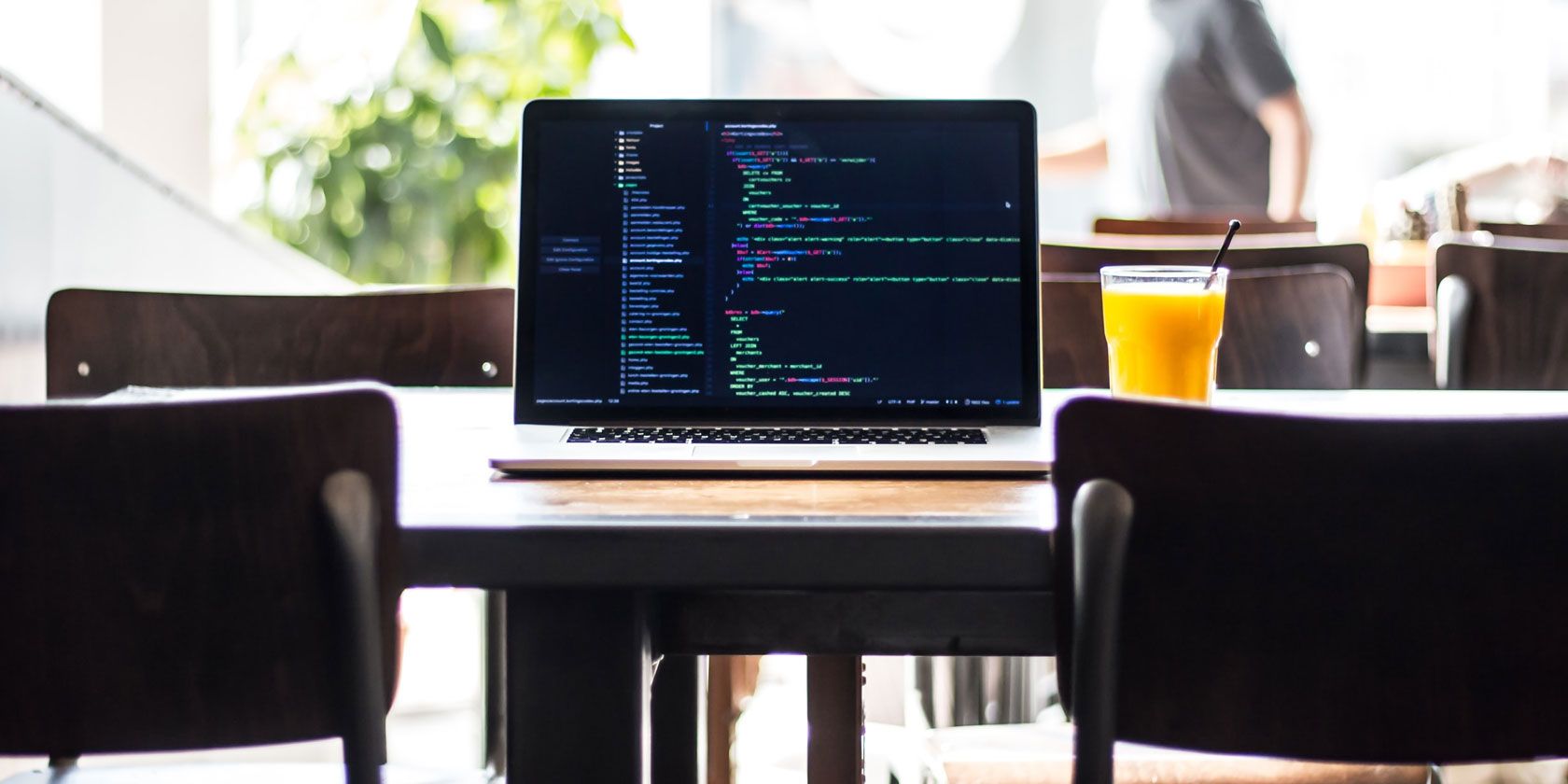
Web developers of all skill levels, from rookie programmers to coding experts, come to see the importance of JavaScript in developing modern websites. JavaScript is so dominant that it is an essential skill to know if you’re going to create apps.
One of the most powerful building blocks built-in to the JavaScript language is arrays. Arrays are commonly found in many programming languages and are useful for storing data.
JavaScript uses arrays but adds useful features known as array methods. There are five you should take a close look at to grow your skills as a developer.
What Are Array Methods?
Array methods are functions built-in to JavaScript that you can apply to your arrays. Each method has a unique function that performs a change or calculation to your array, saving you from needing to code common functions from scratch.
Array methods in JavaScript are ran using a dot-notation attached to your array variable. Since they are just JavaScript functions, they always end with parenthesis which can hold optional arguments. Here is a method attached to a simple array called myArray.
var myArray = [1,2,3];
myArray.pop();
This code would apply a method called pop to the array.
Example Array
For each example let’s use a sample array that we will call myArray, to perform the methods on. Feel free to pull up your console and code along as we go.
var myArray = [2,4,5,7,9,12,14];
These examples will assume you know the foundation of what JavaScript is and how it works. If you don’t that’s okay, we’re all here to learn and grow.
Let’s dig into five powerful methods!
1. Array.push()
What it does: push() takes your array and adds one or more elements to the end of the array, then returns the new length of the array. This method will modify your existing array.
Let’s add the number 20 to the array by running push(), using 20 as an argument.
var myArray = [2,4,5,7,9,12,14];
myArray.push(20);
Let’s see if it worked
console.log(myArray);
[2,4,5,7,9,12,14,20]
Running the push() method on myArray added the value given in the argument into the array. In this case, 20. When you check myArray in the console, you will see the value is now added to the end of the array.
2. Array.concat()
What is does: concat() can merge two or more arrays together into a new array. It does not modify the existing arrays but creates a new one.
Let’s take myArray and merge an array called newArray into it.
var myArray = [2,4,5,7,9,12,14];
var newArray = [1,2,3];
var result = myArray.concat(newArray);
console.log(result);
[2,4,5,7,9,12,14,1,2,3]
This method works wonders when dealing with multiple arrays or values you need to combine, all in one pretty simple step when using variables.
3. Array.join()
What it does: join() takes an array and concatenates the contents of the array, separated by a comma. The result is placed in a string. You can specify a separator if you want to use an alternative to a comma.
Let’s take a look at how this works using myArray. First using the default method with no separator argument, which will use a comma.
var myArray = [2,4,5,7,9,12,14];
myArray.join();
"2,4,5,7,9,12,14"
JavaScript will output a string, with each value in the array separated by a comma. You can use an argument in the function to change the separator. Let’s see it with two arguments: a single space, and a string.
myArray.join(' ');
"2 4 5 7 9 12 14"
myArray.join(' and ');
"2 and 4 and 5 and 7 and 9 and 12 and 14"
The first example is a space, making a string you can easily read.
The second example uses (‘ and ‘), and there are two things to know here.
First, we’re using the word ‘and’ to separate the values. Secondly, there is a space on both sides of the word ‘and’. This is an important thing to keep in mind when using join(). JavaScript reads arguments literally so if this space is left out, everything will be scrunched together (ie. “2and4and5…” etc.) Not a very readable output!
4. Array.forEach()
What it does: forEach() (case sensitive!) performs a function on each item in your array. This function is any function you create, similar to using a “for” loop to apply a function to an array but with much less work to code.
There is a little bit more to forEach() so let’s look at the syntax, then perform a simple function to demonstrate.
myArray.forEach(function(item){
//code
});
We’re using myArray, forEach() is applied with the dot notation. You place the function you wish to use inside of the argument parenthesis, which is function(item) in the example.
Let’s talk about function(item). This is the function executed inside of forEach(), and it has its own argument. We’re calling the argument item. There are two things to know about this argument:
- When forEach() loops over your array, it applies the code to this argument. Think of it as a temporary variable that holds the current element.
- You choose the name of the argument, it can be named anything you want. Typically this would be named something that makes it easier to understand, like “item” or “element”.
With those two things in mind, let’s see a simple example. Let’s add 15 to every value, and have the console show the result.
myArray.forEach(function(item){
console.log(item + 15);
});
We’re using item in this example as the variable, so the function is written to add 15 to each value via item. The console then prints the results. Here’s what it looks like in a Google Chrome console.
The result is every number in the array, but with 15 added to it!
5. Array.map()
What it does: map() performs a function on every element in your array and places the result in a new array.
Running a function on every element sounds like forEach(). The difference here is map() creates a new array when ran. ForEach() does not create a new array automatically, you would have to code a specific function to do so.
Let’s use map() to double the value of every element in myArray, and place them in a new array. You will see the same function(item) syntax for a little more practice.
var myArray = [2,4,5,7,9,12,14];
var doubleArray = myArray.map(function(item){
return item * 2;
});
Let’s check the results in the console.
console.log(doubleArray);
[4,8,10,14,18,24,28]
myArray is unchanged:
console.log(myArray);
[2,4,5,7,9,12,14]
Next Steps in Your JavaScript Journey
Arrays are a powerful part of the JavaScript language, which is why there are so many methods built-in to make your life easier as a developer. The best way to master these five methods is to practice.
As you continue to learn JavaScript, MDN is a great resource for detailed documentation. Get comfortable in the console, then take your skills up a notch with the best JavaScript editors for programmers. Happy coding!
Read the full article: 5 JavaScript Array Methods You Should Master Today
https://ift.tt/34p6ezvvia MakeUseOf
0 comments:
Post a Comment